To restart my career as a technical writer, I chose a light topic. Namely, running applications compiled with new versions of Visual Studio on Windows XP. I didn’t find any prior research on the topic, but I also didn’t search much. There’s no real purpose behind this article, beyond the fact that I wanted to know what could prevent a new application to run on XP. Our target application will be the embedded version of Python 3.7 for x86.
If we try to start any new application on XP, we’ll get an error message informing us that it is not a valid Win32 application. This happens because of some fields in the Optional Header of the Portable Executable.
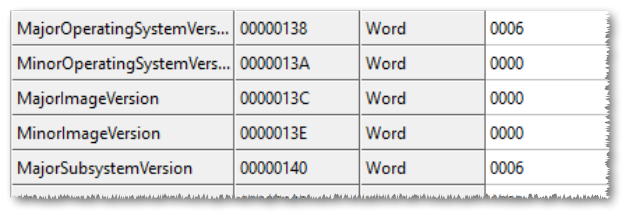
Most of you probably already know that you need to adjust these fields as follows:
MajorOperatingSystemVersion: 5
MinorOperatingSystemVersion: 0
MajorSubsystemVersion: 5
MinorSubsystemVersion: 0
Fortunately, it’s enough to adjust the fields in the executable we want to start (python.exe), there’s no need to adjust the DLLs as well.
If we try run the application now, we’ll get an error message due to a missing API in kernel32. So let’s turn our attention to the imports.
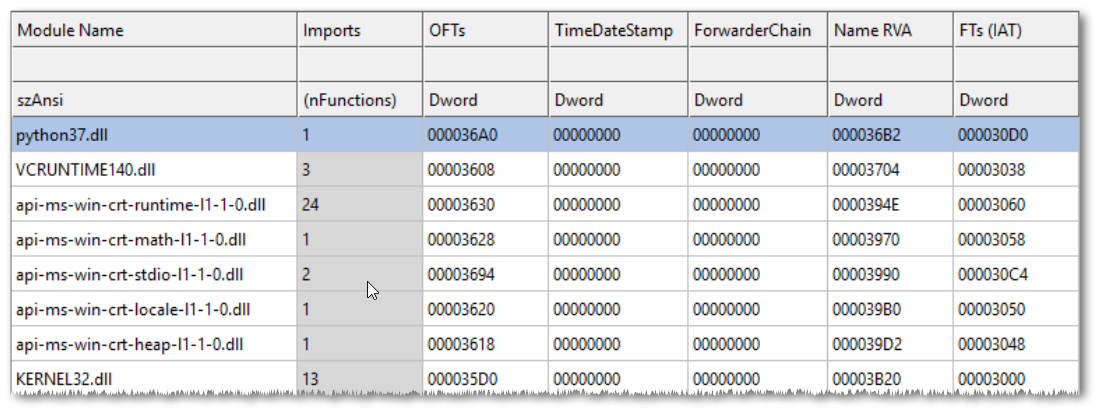
We have a missing vcruntime140.dll, then a bunch of “api-ms-win-*” DLLs, then only python37.dll and kernel32.dll.
The first thing which comes to mind is that in new applications we often find these “api-ms-win-*” DLLs. If we search for the prefix in the Windows directory, we’ll find a directory both in System32 and SysWOW64 called “downlevel”, which contains a huge list of these DLLs.
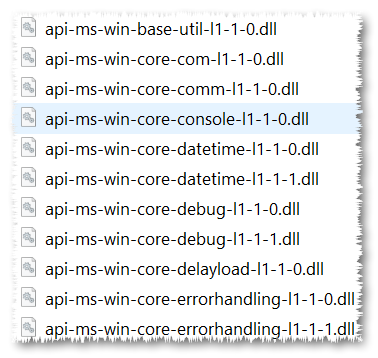
As we’ll see later, these DLLs aren’t actually used, but if we open one with a PE viewer, we’ll see that it contains exclusively forwarders to APIs contained in the usual suspects such as kernel32, kernelbase, user32 etc.
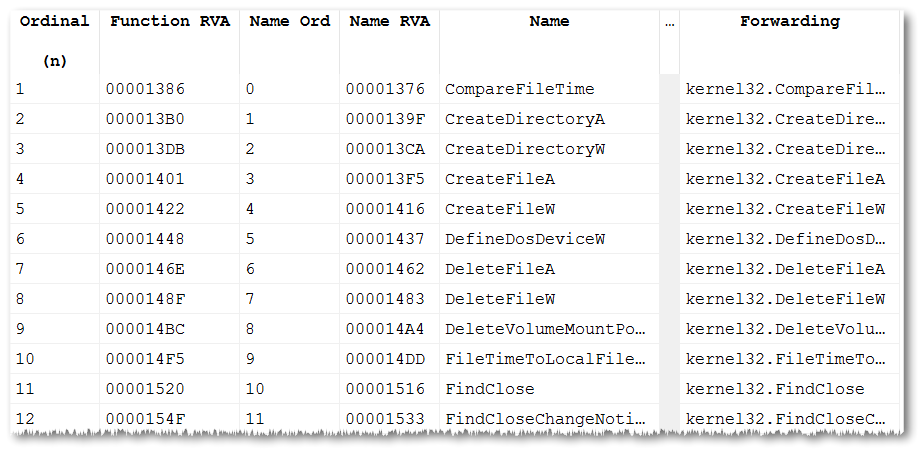
There’s a MSDN page documenting these DLLs.
Interestingly, in the downlevel directory we can’t find any of the files imported by python.exe. These DLLs actually expose C runtime APIs like strlen, fopen, exit and so on.
If we don’t have any prior knowledge on the topic and just do a string search inside the Windows directory for such a DLL name, we’ll find a match in C:\Windows\System32\apisetschema.dll. This DLL is special as it contains a .apiset section, whose data can easily be identified as some sort of format for mapping “api-ms-win-*” names to others.
Offset 0 1 2 3 4 5 6 7 8 9 A B C D E F Ascii
00013AC0 C8 3A 01 00 20 00 00 00 73 00 74 00 6F 00 72 00 .:......s.t.o.r.
00013AD0 61 00 67 00 65 00 75 00 73 00 61 00 67 00 65 00 a.g.e.u.s.a.g.e.
00013AE0 2E 00 64 00 6C 00 6C 00 65 00 78 00 74 00 2D 00 ..d.l.l.e.x.t.-.
00013AF0 6D 00 73 00 2D 00 77 00 69 00 6E 00 2D 00 73 00 m.s.-.w.i.n.-.s.
00013B00 78 00 73 00 2D 00 6F 00 6C 00 65 00 61 00 75 00 x.s.-.o.l.e.a.u.
00013B10 74 00 6F 00 6D 00 61 00 74 00 69 00 6F 00 6E 00 t.o.m.a.t.i.o.n.
00013B20 2D 00 6C 00 31 00 2D 00 31 00 2D 00 30 00 00 00 -.l.1.-.1.-.0...
00013B30 00 00 00 00 00 00 00 00 00 00 00 00 44 3B 01 00 ............D;..
00013B40 0E 00 00 00 73 00 78 00 73 00 2E 00 64 00 6C 00 ....s.x.s...d.l.
00013B50 6C 00 00 00 65 00 78 00 74 00 2D 00 6D 00 73 00 l...e.x.t.-.m.s.
Searching on the web, the first resource I found on this topic were two articles on the blog of Quarkslab (Part 1 and Part 2). However, I quickly figured that, while useful, they were too dated to provide me with up-to-date structures to parse the data. In fact, the second article shows a version number of 2 and at the time of my writing the version number is 6.
Offset 0 1 2 3 4 5 6 7 8 9 A B C D E F Ascii
00000000 06 00 00 00 ....
Just for completeness, after the publication of the current article, I was made aware of an article by deroko about the topic predating those of Quarkslab.
Anyway, I searched some more and found a code snippet by Alex Ionescu and Pavel Yosifovich in the repository of Windows Internals. I took the following structures from there.
typedef struct _API_SET_NAMESPACE {
ULONG Version;
ULONG Size;
ULONG Flags;
ULONG Count;
ULONG EntryOffset;
ULONG HashOffset;
ULONG HashFactor;
} API_SET_NAMESPACE, *PAPI_SET_NAMESPACE;
typedef struct _API_SET_HASH_ENTRY {
ULONG Hash;
ULONG Index;
} API_SET_HASH_ENTRY, *PAPI_SET_HASH_ENTRY;
typedef struct _API_SET_NAMESPACE_ENTRY {
ULONG Flags;
ULONG NameOffset;
ULONG NameLength;
ULONG HashedLength;
ULONG ValueOffset;
ULONG ValueCount;
} API_SET_NAMESPACE_ENTRY, *PAPI_SET_NAMESPACE_ENTRY;
typedef struct _API_SET_VALUE_ENTRY {
ULONG Flags;
ULONG NameOffset;
ULONG NameLength;
ULONG ValueOffset;
ULONG ValueLength;
} API_SET_VALUE_ENTRY, *PAPI_SET_VALUE_ENTRY;
The data starts with a API_SET_NAMESPACE structure.
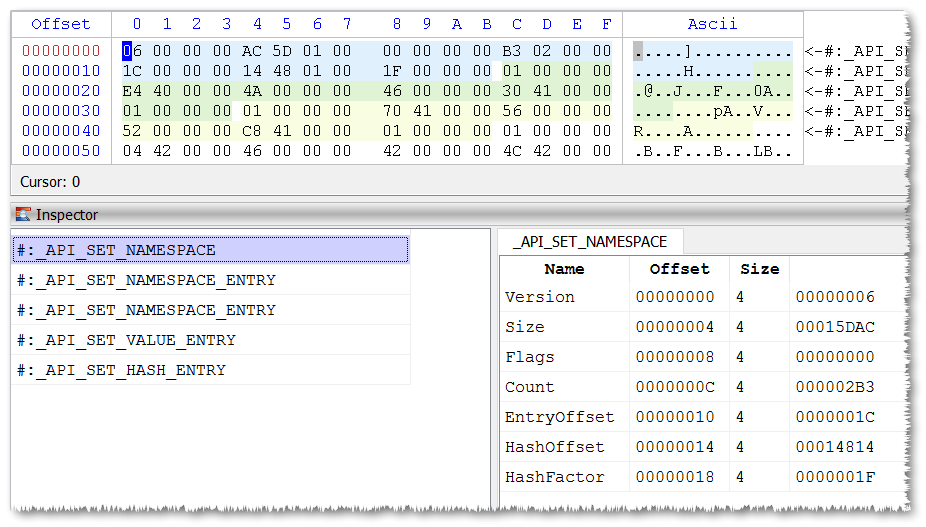
Count specifies the number of API_SET_NAMESPACE_ENTRY and API_SET_HASH_ENTRY structures. EntryOffset points to the start of the array of API_SET_NAMESPACE_ENTRY structures, which in our case comes exactly after API_SET_NAMESPACE.
Every API_SET_NAMESPACE_ENTRY points to the name of the “api-ms-win-*” DLL via the NameOffset field, while ValueOffset and ValueCount specify the position and count of API_SET_VALUE_ENTRY structures. The API_SET_VALUE_ENTRY structure yields the resolution values (e.g. kernel32.dll, kernelbase.dll) for the given “api-ms-win-*” DLL.
With this information we can already write a small script to map the new names to the actual DLLs.
import os
from Pro.Core import *
from Pro.PE import *
def main():
c = createContainerFromFile("C:\\Windows\\System32\\apisetschema.dll")
pe = PEObject()
if not pe.Load(c):
print("couldn't load apisetschema.dll")
return
sect = pe.SectionHeaders()
nsects = sect.Count()
d = None
for i in range(nsects):
if sect.Bytes(0) == b".apiset\x00":
cs = pe.SectionData(i)[0]
d = CFFObject()
d.Load(cs)
break
sect = sect.Add(1)
if not d:
print("could find .apiset section")
return
n, ret = d.ReadUInt32(12)
offs, ret = d.ReadUInt32(16)
for i in range(n):
name_offs, ret = d.ReadUInt32(offs + 4)
name_size, ret = d.ReadUInt32(offs + 8)
name = d.Read(name_offs, name_size).decode("utf-16")
line = str(i) + ") " + name + " ->"
values_offs, ret = d.ReadUInt32(offs + 16)
value_count, ret = d.ReadUInt32(offs + 20)
for j in range(value_count):
vname_offs, ret = d.ReadUInt32(values_offs + 12)
vname_size, ret = d.ReadUInt32(values_offs + 16)
vname = d.Read(vname_offs, vname_size).decode("utf-16")
line += " " + vname
values_offs += 20
offs += 24
print(line)
main()
This code can be executed with Cerbero Profiler from command line as “cerpro.exe -r apisetschema.py”. These are the first lines of the produced output:
0) api-ms-onecoreuap-print-render-l1-1-0 -> printrenderapihost.dll
1) api-ms-onecoreuap-settingsync-status-l1-1-0 -> settingsynccore.dll
2) api-ms-win-appmodel-identity-l1-2-0 -> kernel.appcore.dll
3) api-ms-win-appmodel-runtime-internal-l1-1-3 -> kernel.appcore.dll
4) api-ms-win-appmodel-runtime-l1-1-2 -> kernel.appcore.dll
5) api-ms-win-appmodel-state-l1-1-2 -> kernel.appcore.dll
6) api-ms-win-appmodel-state-l1-2-0 -> kernel.appcore.dll
7) api-ms-win-appmodel-unlock-l1-1-0 -> kernel.appcore.dll
8) api-ms-win-base-bootconfig-l1-1-0 -> advapi32.dll
9) api-ms-win-base-util-l1-1-0 -> advapi32.dll
10) api-ms-win-composition-redirection-l1-1-0 -> dwmredir.dll
11) api-ms-win-composition-windowmanager-l1-1-0 -> udwm.dll
12) api-ms-win-core-apiquery-l1-1-0 -> ntdll.dll
13) api-ms-win-core-appcompat-l1-1-1 -> kernelbase.dll
14) api-ms-win-core-appinit-l1-1-0 -> kernel32.dll kernelbase.dll
...
Going back to API_SET_NAMESPACE, its field HashOffset points to an array of API_SET_HASH_ENTRY structures. These structures, as we’ll see in a moment, are used by the Windows loader to quickly index a “api-ms-win-*” DLL name. The Hash field is effectively the hash of the name, calculated by taking into consideration both HashFactor and HashedLength, while Index points to the associated API_SET_NAMESPACE_ENTRY entry.
The code which does the hashing is inside the function LdrpPreprocessDllName in ntdll:
77EA1DAC mov ebx, dword ptr [ebx + 0x18] ; HashFactor in ebx
77EA1DAF mov esi, eax ; esi = dll name length
77EA1DB1 movzx eax, word ptr [edx] ; one unicode character into eax
77EA1DB4 lea ecx, dword ptr [eax - 0x41] ; ecx = character - 0x41
77EA1DB7 cmp cx, 0x19 ; compare to 0x19
77EA1DBB jbe 0x77ea2392 ; if below or equal, bail out
77EA1DC1 mov ecx, ebx ; ecx = HashFactor
77EA1DC3 movzx eax, ax
77EA1DC6 imul ecx, edi ; ecx *= edi
77EA1DC9 add edx, 2 ; edx += 2
77EA1DCC add ecx, eax ; ecx += eax
77EA1DCE mov edi, ecx ; edi = ecx
77EA1DD0 sub esi, 1 ; len -= 1
77EA1DD3 jne 0x77ea1db1 ; if not zero repeat from 77EA1DB1
Or more simply in C code:
const char *p = dllname;
int HashedLength = 0x23;
int HashFactor = 0x1F;
int Hash = 0;
for (int i = 0; i < HashedLength; i++, p++)
Hash = (Hash * HashFactor) + *p;
As a practical example, let's take the DLL name "api-ms-win-core-processthreads-l1-1-2.dll". Its hash would be 0x445B4DF3. If we find its matching API_SET_HASH_ENTRY entry, we'll have the Index to the associated API_SET_NAMESPACE_ENTRY structure.
Offset 0 1 2 3 4 5 6 7 8 9 A B C D E F Ascii
00014DA0 F3 4D 5B 44 .M[D
00014DB0 5B 00 00 00 [...
So, 0x5b (or 91) is the index. By going back to the output of mappings, we can see that it matches.
91) api-ms-win-core-processthreads-l1-1-3 -> kernel32.dll kernelbase.dll
By inspecting the same output, we can also notice that all C runtime DLLs are resolved to ucrtbase.dll.
167) api-ms-win-crt-conio-l1-1-0 -> ucrtbase.dll
168) api-ms-win-crt-convert-l1-1-0 -> ucrtbase.dll
169) api-ms-win-crt-environment-l1-1-0 -> ucrtbase.dll
170) api-ms-win-crt-filesystem-l1-1-0 -> ucrtbase.dll
171) api-ms-win-crt-heap-l1-1-0 -> ucrtbase.dll
172) api-ms-win-crt-locale-l1-1-0 -> ucrtbase.dll
173) api-ms-win-crt-math-l1-1-0 -> ucrtbase.dll
174) api-ms-win-crt-multibyte-l1-1-0 -> ucrtbase.dll
175) api-ms-win-crt-private-l1-1-0 -> ucrtbase.dll
176) api-ms-win-crt-process-l1-1-0 -> ucrtbase.dll
177) api-ms-win-crt-runtime-l1-1-0 -> ucrtbase.dll
178) api-ms-win-crt-stdio-l1-1-0 -> ucrtbase.dll
179) api-ms-win-crt-string-l1-1-0 -> ucrtbase.dll
180) api-ms-win-crt-time-l1-1-0 -> ucrtbase.dll
181) api-ms-win-crt-utility-l1-1-0 -> ucrtbase.dll
I was already resigned at having to figure out how to support the C runtime on XP, when I noticed that Microsoft actually supports the deployment of the runtime on it. The following excerpt from MSDN says as much:
If you currently use the VCRedist (our redistributable package files), then things will just work for you as they did before. The Visual Studio 2015 VCRedist package includes the above mentioned Windows Update packages, so simply installing the VCRedist will install both the Visual C++ libraries and the Universal CRT. This is our recommended deployment mechanism. On Windows XP, for which there is no Universal CRT Windows Update MSU, the VCRedist will deploy the Universal CRT itself.
Which means that on Windows editions coming after XP the support is provided via Windows Update, but on XP we have to deploy the files ourselves. We can find the files to deploy inside C:\Program Files (x86)\Windows Kits\10\Redist\ucrt\DLLs. This path contains three sub-directories: x86, x64 and arm. We're obviously interested in the x86 one. The files contained in it are many (42), apparently the most common "api-ms-win-*" DLLs and ucrtbase.dll. We can deploy those files onto XP to make our application work. We are still missing the vcruntime140.dll, but we can take that DLL from the Visual C++ installation. In fact, that DLL is intended to be deployed, while the Universal CRT (ucrtbase.dll) is intended to be part of the Windows system.
This satisfies our dependencies in terms of DLLs. However, Windows introduced many new APIs over the years which aren't present on XP. So I wrote a script to test the compatibility of an application by checking the imported APIs against the API exported by the DLLs on XP. The command line for it is "cerpro.exe -r xpcompat.py application_path". It will check all the PE files in the specified directory.
import os, sys
from Pro.Core import *
from Pro.PE import *
xp_system32 = "C:\\Users\\Admin\\Desktop\\system32"
apisetschema = { "OMITTED FOR BREVITY" }
cached_apis = {}
missing_result = {}
def getAPIs(dllpath):
apis = {}
c = createContainerFromFile(dllpath)
dll = PEObject()
if not dll.Load(c):
print("error: couldn't load dll")
return apis
ordbase = dll.ExportDirectory().Num("Base")
functions = dll.ExportDirectoryFunctions()
names = dll.ExportDirectoryNames()
nameords = dll.ExportDirectoryNameOrdinals()
n = functions.Count()
it = functions.iterator()
for x in range(n):
func = it.next()
ep = func.Num(0)
if ep == 0:
continue
apiord = str(ordbase + x)
n2 = nameords.Count()
it2 = nameords.iterator()
name_found = False
for y in range(n2):
no = it2.next()
if no.Num(0) == x:
name = names.At(y)
offs = dll.RvaToOffset(name.Num(0))
name, ret = dll.ReadUInt8String(offs, 500)
apiname = name.decode("ascii")
apis[apiname] = apiord
apis[apiord] = apiname
name_found = True
break
if not name_found:
apis[apiord] = apiord
return apis
def checkMissingAPIs(pe, ndescr, dllname, xpdll_apis):
ordfl = pe.ImportOrdinalFlag()
ofts = pe.ImportThunks(ndescr)
it = ofts.iterator()
while it.hasNext():
ft = it.next().Num(0)
if (ft & ordfl) != 0:
name = str(ft ^ ordfl)
else:
offs = pe.RvaToOffset(ft)
name, ret = pe.ReadUInt8String(offs + 2, 400)
if not ret:
continue
name = name.decode("ascii")
if not name in xpdll_apis:
print(" ", "missing:", name)
temp = missing_result.get(dllname, set())
temp.add(name)
missing_result[dllname] = temp
def verifyXPCompatibility(fname):
print("file:", fname)
c = createContainerFromFile(fname)
pe = PEObject()
if not pe.Load(c):
return
it = pe.ImportDescriptors().iterator()
ndescr = -1
while it.hasNext():
descr = it.next()
ndescr += 1
offs = pe.RvaToOffset(descr.Num("Name"))
name, ret = pe.ReadUInt8String(offs, 400)
if not ret:
continue
name = name.decode("ascii").lower()
if not name.endswith(".dll"):
continue
fwdlls = apisetschema.get(name[:-4], [])
if len(fwdlls) == 0:
print(" ", name)
else:
fwdll = fwdlls[0]
print(" ", name, "->", fwdll)
name = fwdll
if name == "ucrtbase.dll":
continue
xpdll_path = os.path.join(xp_system32, name)
if not os.path.isfile(xpdll_path):
continue
if not name in cached_apis:
cached_apis[name] = getAPIs(xpdll_path)
checkMissingAPIs(pe, ndescr, name, cached_apis[name])
print()
def main():
if os.path.isfile(sys.argv[1]):
verifyXPCompatibility(sys.argv[1])
else:
files = [os.path.join(dp, f) for dp, dn, fn in os.walk(sys.argv[1]) for f in fn]
for fname in files:
with open(fname, "rb") as f:
if f.read(2) == b"MZ":
verifyXPCompatibility(fname)
# summary
n = 0
print("\nsummary:")
for rdll, rapis in missing_result.items():
print(" ", rdll)
for rapi in rapis:
print(" ", "missing:", rapi)
n += 1
print("total of missing APIs:", str(n))
main()
I had to omit the contents of the apisetschema global variable for the sake of brevity. You can download the full script from here. The system32 directory referenced in the code is the one of Windows XP, which I copied to my desktop.
And here are the relevant excerpts from the output:
file: python-3.7.0-embed-win32\python37.dll
version.dll
shlwapi.dll
ws2_32.dll
kernel32.dll
missing: GetFinalPathNameByHandleW
missing: InitializeProcThreadAttributeList
missing: UpdateProcThreadAttribute
missing: DeleteProcThreadAttributeList
missing: GetTickCount64
advapi32.dll
vcruntime140.dll
api-ms-win-crt-runtime-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-math-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-locale-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-string-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-stdio-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-convert-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-time-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-environment-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-process-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-heap-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-conio-l1-1-0.dll -> ucrtbase.dll
api-ms-win-crt-filesystem-l1-1-0.dll -> ucrtbase.dll
[...]
file: python-3.7.0-embed-win32\_socket.pyd
ws2_32.dll
missing: inet_ntop
missing: inet_pton
kernel32.dll
python37.dll
vcruntime140.dll
api-ms-win-crt-runtime-l1-1-0.dll -> ucrtbase.dll
[...]
summary:
kernel32.dll
missing: InitializeProcThreadAttributeList
missing: GetTickCount64
missing: GetFinalPathNameByHandleW
missing: UpdateProcThreadAttribute
missing: DeleteProcThreadAttributeList
ws2_32.dll
missing: inet_pton
missing: inet_ntop
total of missing APIs: 7
We're missing 5 APIs from kernel32.dll and 2 from ws2_32.dll, but the Winsock APIs are imported just by _socket.pyd, a module which is loaded only when a network operation is performed by Python. So, in theory, we can focus our efforts on the missing kernel32 APIs for now.
My plan was to create a fake kernel32.dll, called xernel32.dll, containing forwarders for most APIs and real implementations only for the missing ones. Here's a script to create C++ files containing forwarders for all APIs of common DLLs on Windows 10:
import os, sys
from Pro.Core import *
from Pro.PE import *
xpsys32path = "C:\\Users\\Admin\\Desktop\\system32"
sys32path = "C:\\Windows\\SysWOW64"
def getAPIs(dllpath):
pass # same code as above
def isOrdinal(i):
try:
int(i)
return True
except:
return False
def createShadowDll(name):
xpdllpath = os.path.join(xpsys32path, name + ".dll")
xpapis = getAPIs(xpdllpath)
dllpath = os.path.join(sys32path, name + ".dll")
apis = sorted(getAPIs(dllpath).keys())
if len(apis) != 0:
with open(name + ".cpp", "w") as f:
f.write("#include \n\n")
for a in apis:
comment = " // XP" if a in xpapis else ""
if not isOrdinal(a):
f.write("#pragma comment(linker, \"/export:" + a + "=" + name + "." + a + "\")" + comment + "\n")
#
print("created", name + ".cpp")
def main():
dlls = ("advapi32", "comdlg32", "gdi32", "iphlpapi", "kernel32", "ole32", "oleaut32", "shell32", "shlwapi", "user32", "uxtheme", "ws2_32")
for dll in dlls:
createShadowDll(dll)
main()
It creates files like the following kernel32.cpp:
#include
#pragma comment(linker, "/export:AcquireSRWLockExclusive=kernel32.AcquireSRWLockExclusive")
#pragma comment(linker, "/export:AcquireSRWLockShared=kernel32.AcquireSRWLockShared")
#pragma comment(linker, "/export:ActivateActCtx=kernel32.ActivateActCtx") // XP
#pragma comment(linker, "/export:ActivateActCtxWorker=kernel32.ActivateActCtxWorker")
#pragma comment(linker, "/export:AddAtomA=kernel32.AddAtomA") // XP
#pragma comment(linker, "/export:AddAtomW=kernel32.AddAtomW") // XP
#pragma comment(linker, "/export:AddConsoleAliasA=kernel32.AddConsoleAliasA") // XP
#pragma comment(linker, "/export:AddConsoleAliasW=kernel32.AddConsoleAliasW") // XP
#pragma comment(linker, "/export:AddDllDirectory=kernel32.AddDllDirectory")
[...]
The comment on the right ("// XP") indicates whether the forwarded API is present on XP or not. We can provide real implementations exclusively for the APIs we want. The Windows loader doesn't care whether we forward functions which don't exist as long as they aren't imported.
The APIs we need to support are the following:
GetTickCount64: I just called GetTickCount, not really important
GetFinalPathNameByHandleW: took the implementation from Wine, but had to adapt it slightly
InitializeProcThreadAttributeList: took the implementation from Wine
UpdateProcThreadAttribute: same
DeleteProcThreadAttributeList: same
I have to be grateful to the Wine project here, as it provided useful implementations, which saved me the effort.
I called the attempt at a support runtime for older Windows versions "XP Time Machine Runtime" and you can find the repository here. I compiled it with Visual Studio 2013 and cmake.
So that we have now our xernel32.dll, the only thing we have to do is to rename the imported DLL inside python37.dll.
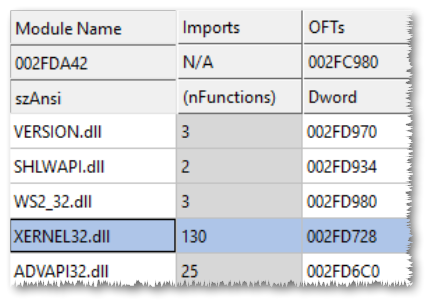
Let's try to start python.exe.
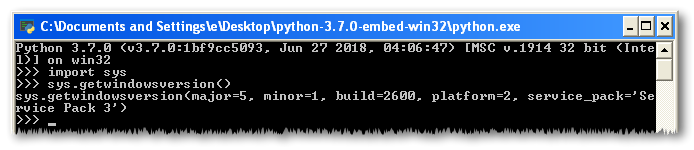
Awesome.
Of course, we're still not completely done, as we didn't implement the missing Winsock APIs, but perhaps this and some more could be the content of a second part to this article.
Great analysis!
I’ll have to re-read it a couple of times to understand the details: it’s really an awesome work (you had to spend quite some time to put the pieces together, do researches, debug windows imports loading, write and debug Cerbero scripts, ecc.).
Thank you for sharing your knowledge!
And, of course, welcome back ! You rock!
Best Regards,
Tony
Thanks Tony!
Really glad you liked it π
This is awesome !
Since I stuck with XP to access an old ERP via odbc, (Navision, codbc driver),
this gives me some hope to be able to lift my XP Gateway to 3.7 ….
Great work !
yours sincerely
Robert
Thank you, Robert! π
You are a warrior without fears indeed π
Congratulations for this article! I still feel a bit dizzy after reading it!
Thank you, Denes! Much appreciate it π
Without any intention to advertise, let me show you this:
http://deneskellner.com/sw/rapidexe
This is why I was looking for a solution to run Python on XP. It seems too much pain in the basement now; but if you find a solution to extend this tool with something-XP-ish, I’d be glad to add it as a new engine. Aaand, let me know what you think about it anyway!
(It’s a very simple tool but I really enjoy using it)
Hello Denes,
I didn’t try it, but as a concept it’s clearly useful. Especially to deploy scripts to non-technical users. Maybe sooner or later I’ll wrap up my writing on XP and also make it simpler to integrate. π
Not a coder and only understood about a third of it (wandered in looking for a way to run Vista/Win7 apps on XP, or at least XP64), but I’m quite impressed by your detective skills. Bravo!
Thank you, Reziac! π
This article is really a pleasure to read, got some questions though, I see you use CFF explorer for many of the shots, which software is used to create this [one](https://ntcore.com/wp-content/uploads/2018/xptm/forwarders.png)?
Also, you say ‘weβll find a directory both in System32 and SysWOW64 called βdownlevelβ, which contains a huge list of these DLLs.’. I’ve installed a fresh windows xp as a virtual-machine, installed vcredist_x86.exe from VisualStudio2015 and I still don’t see neither that `downlevel` directory nor `apisetschema.dll`, could you clarify here?
I see `apisetschema.dll` is living on my devbox (windows7) though… i assume this is not coming from any visual studio redistributable package?
All in all, this is really high quality article and I miss more articles like this one across the whole internet π
ps. i was trying to build windows xp apps by using nuitka so this article definitely will help me in the task hehe π
Hello BPL,
thank you, I’m glad you enjoyed the article. I always try to write articles which are a bit different than those usually found across the internet. So hearing that this is appreciated is rewarding. π
The software used for the screen-shot is the following: https://store.cerbero.io/profiler/download/
It’s a commercial application I wrote, but it has a free trial.
It’s also the same application used to execute the Python code. (all those Pro headers are inside the application). The syntax to execute the scripts is explained in the article: search for cerpro.exe -r.
The downlevel directory is present on Windows 10. I don’t know if it’s present on other versions of Windows. But those DLLs aren’t used. When you install the redist the DLLs can be found under: C:\Program Files (x86)\Windows Kits\10\Redist\ucrt\DLLs. Same for apisetschema.dll. It’s a DLL in Windows 10, but it’s present also on other version of Windows (not XP). You don’t need that DLL. It just contains the mappings to establish where the APIs are actually located.
I hope this helps!
Hello dpistelli,
first of all I want to thank you for this detailed and thorough analysis.
I am fairly new to this topic, which is why I’ll need to read it a few more times to get everything, but I have a very simple question, because I am right now facing the problem
of running an executable on XP because of a missing API.
As far as I understood, after running the above shown script, I get all of the cpp files that are also in your Github repository…
If I now want to rename the important dll inside my application, I have two questions:
1) it is just an .exe (originally written in python) so how do I get to the point shown in your penultimate screenshot where I change the dll
2) do I have to create a dll (I’ll just also call it “xernel.dll”) file and just copy the content of the kernel32.cpp into it in order for it to function?
Thanks in advance and I hope to hear from you soon!
Hello ovi,
thank you, glad you liked it!
well the files on github have already few of the missing APIs added. So I recommend you get the files from GitHub.
1) It probably just extracts the Python files, you need to get to the point where it does it. Check perhaps with a tool like Process Monitor where it extracts the files.
2) You need to compile a xernel32 dll with the missing API, yes. No need to copy the entire kernel32, just implement the one missing API inside xernel32, the other APIs will be just forwarded.
Hope this helps!
Cheers
Hello Erik,
So, finally… Have you been using your hacked python 3.7 on Windows XP ?
Hello Adam,
Yes, in fact I deploy it in my Cerbero Suite in order to support Windows XP. π
how can i run python3.7 on xp
Well, the answer is in the article. It’s not so easy. The best way would be if the developers of Python would allow for it. π
This is an inspiring article for a newbie like me. I would like to compile xernel32.dll myself, but I assume that if it is compiled with Visual Studio 2013 the target machine has to have the 2013 runtimes installed. Am I right in assuming that the #pragma comment feature belongs to MSVC, and that I could not compile xernel32.dll with the MinGW C compiler to avoid the need for VC++ runtimes?
Also an example of the the commands you could use for compiling xernel32.dll using the MinGW C++ compiler would be helpful because I already have MinGW installed, and don’t want to download and install Visual Studio 2013 for a single task.
Thank you, Maurice.
While it is indeed compiled with VS2013, the target does not need the runtime installed. You can statically link against the runtime. That’s exactly what the following cmake line does:
string(REPLACE “/MD” “/MT” ${COMPFLAG} “${${COMPFLAG}}”)
So the runtime is not an issue. As for MinGW, pragma statements are often supported by other compilers. Unfortunately, I don’t know if MinGW supports the needed ones as I don’t use MinGW.
Great writeup. Clear, concise and to the point.
It’s very educational and altough I’m not a programmer, it’s helpful information on how things work and examples on workarounds for Windows XP.
Thank you!